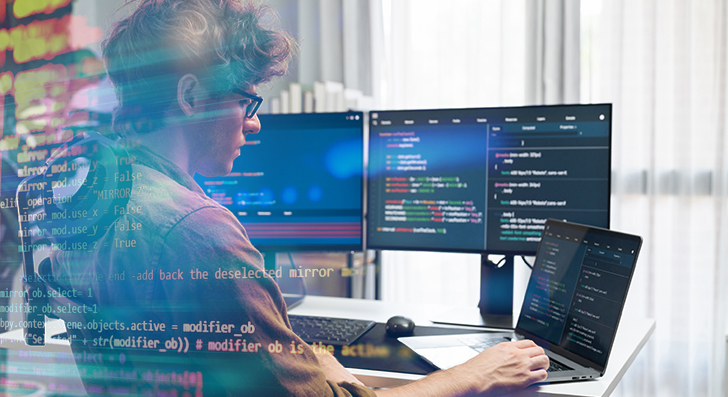
Scalability usually means your software can handle advancement—additional end users, much more data, and more targeted visitors—without the need of breaking. As being a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and useful guidebook that can assist you begin by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later on—it ought to be element within your prepare from the beginning. Many apps fail if they develop speedy due to the fact the original style and design can’t cope with the extra load. For a developer, you have to Assume early about how your technique will behave under pressure.
Commence by building your architecture being flexible. Prevent monolithic codebases exactly where anything is tightly connected. As an alternative, use modular style and design or microservices. These patterns split your application into smaller sized, unbiased components. Each individual module or support can scale By itself without having influencing The entire method.
Also, consider your databases from working day a single. Will it have to have to handle 1,000,000 buyers or perhaps a hundred? Choose the suitable variety—relational or NoSQL—according to how your details will expand. Plan for sharding, indexing, and backups early, even if you don’t will need them still.
Yet another important place is to prevent hardcoding assumptions. Don’t compose code that only performs less than current circumstances. Give thought to what would take place In case your user base doubled tomorrow. Would your app crash? Would the database slow down?
Use style and design styles that support scaling, like information queues or party-driven programs. These support your app manage a lot more requests without having acquiring overloaded.
Once you Construct with scalability in mind, you're not just getting ready for success—you're reducing upcoming problems. A very well-planned program is easier to take care of, adapt, and grow. It’s improved to arrange early than to rebuild later on.
Use the correct Database
Choosing the right databases can be a crucial A part of developing scalable applications. Not all databases are crafted the exact same, and using the Incorrect you can sluggish you down or perhaps cause failures as your application grows.
Start out by comprehension your information. Can it be very structured, like rows inside a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great match. These are powerful with interactions, transactions, and consistency. In addition they help scaling procedures like read through replicas, indexing, and partitioning to handle additional site visitors and details.
Should your info is a lot more flexible—like person activity logs, product or service catalogs, or documents—look at a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more quickly.
Also, think about your examine and write designs. Are you presently performing numerous reads with much less writes? Use caching and browse replicas. Are you dealing with a significant write load? Explore databases which can deal with substantial produce throughput, or perhaps function-dependent information storage programs like Apache Kafka (for momentary data streams).
It’s also intelligent to Consider forward. You might not have to have Sophisticated scaling functions now, but picking a databases that supports them means you won’t want to change later on.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information according to your entry designs. And usually keep track of database efficiency while you expand.
In a nutshell, the best databases is dependent upon your application’s framework, pace requires, And exactly how you hope it to mature. Choose time to select correctly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Quick code is vital to scalability. As your app grows, each small hold off provides up. Badly composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you Make successful logic from the beginning.
Start off by creating clean, simple code. Avoid repeating logic and take away everything needless. Don’t choose the most advanced Resolution if a simple 1 works. Keep the features short, centered, and easy to check. Use profiling instruments to discover bottlenecks—places wherever your code will take too long to operate or makes use of too much memory.
Following, take a look at your databases queries. These frequently gradual factors down greater than the code alone. Make certain Each individual question only asks for the information you truly want. Stay clear of Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And keep away from doing too many joins, In particular throughout large tables.
In case you notice the identical data currently being asked for repeatedly, use caching. Keep the results temporarily working with tools like Redis or Memcached therefore you don’t need to repeat high-priced functions.
Also, batch your databases operations once you can. In place of updating a row one after the click here other, update them in groups. This cuts down on overhead and helps make your application extra effective.
Remember to test with big datasets. Code and queries that operate great with a hundred records may well crash whenever they have to manage one million.
To put it briefly, scalable applications are fast apps. Keep your code restricted, your queries lean, and use caching when wanted. These ways help your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more end users plus much more website traffic. If every thing goes by way of one particular server, it can promptly turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these instruments support maintain your app quick, stable, and scalable.
Load balancing spreads incoming targeted traffic across several servers. Rather than 1 server performing all the work, the load balancer routes buyers to distinctive servers based upon availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can mail visitors to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused immediately. When people request exactly the same information yet again—like a product webpage or perhaps a profile—you don’t really need to fetch it through the database when. It is possible to serve it with the cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores details in memory for quickly accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static files near to the person.
Caching lowers database load, enhances velocity, and helps make your application a lot more efficient.
Use caching for things that don’t alter generally. And usually ensure that your cache is updated when facts does alter.
Briefly, load balancing and caching are simple but strong tools. Collectively, they assist your application manage additional customers, remain fast, and Recuperate from complications. If you plan to improve, you need the two.
Use Cloud and Container Instruments
To build scalable applications, you may need applications that let your app increase conveniently. That’s where cloud platforms and containers are available in. They offer you flexibility, decrease setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess long term capability. When targeted traffic boosts, you are able to include a lot more assets with just a couple clicks or routinely applying vehicle-scaling. When traffic drops, you are able to scale down to save money.
These platforms also supply providers like managed databases, storage, load balancing, and safety instruments. You could focus on building your application as an alternative to taking care of infrastructure.
Containers are One more essential Device. A container deals your app and everything it needs to operate—code, libraries, options—into a single unit. This makes it easy to move your application involving environments, from the laptop computer to your cloud, with no surprises. Docker is the most well-liked Instrument for this.
Once your application employs several containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and Restoration. If a single component of your application crashes, it restarts it instantly.
Containers also make it straightforward to individual areas of your app into providers. You can update or scale sections independently, which can be perfect for functionality and reliability.
To put it briefly, making use of cloud and container tools signifies you are able to scale rapid, deploy very easily, and Get better swiftly when complications come about. If you want your application to develop with out boundaries, start employing these tools early. They preserve time, reduce chance, and help you remain centered on building, not repairing.
Observe Every thing
When you don’t monitor your application, you gained’t know when matters go Incorrect. Checking allows you see how your application is undertaking, spot concerns early, and make greater conclusions as your app grows. It’s a important Section of making scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk House, and response time. These tell you how your servers and solutions are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Keep an eye on how long it will take for customers to load webpages, how often mistakes come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or maybe a assistance goes down, you'll want to get notified straight away. This allows you deal with difficulties rapid, generally in advance of end users even observe.
Monitoring can also be helpful when you make changes. For those who deploy a different attribute and see a spike in errors or slowdowns, you could roll it again ahead of it leads to actual injury.
As your application grows, website traffic and info improve. Without the need of checking, you’ll skip indications of difficulties until finally it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring allows you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your procedure and ensuring it really works very well, even under pressure.
Closing Ideas
Scalability isn’t only for large providers. Even tiny applications want a solid foundation. By coming up with very carefully, optimizing correctly, and using the appropriate tools, it is possible to build apps that improve smoothly with no breaking stressed. Begin smaller, Believe massive, and Establish wise.